To prepopulate questions on an inspection, you should begin by adding the Inspection Response Id field onto the Work Order Service Task form. This field can be hidden or displayed, as needed, but it must be included on the form.
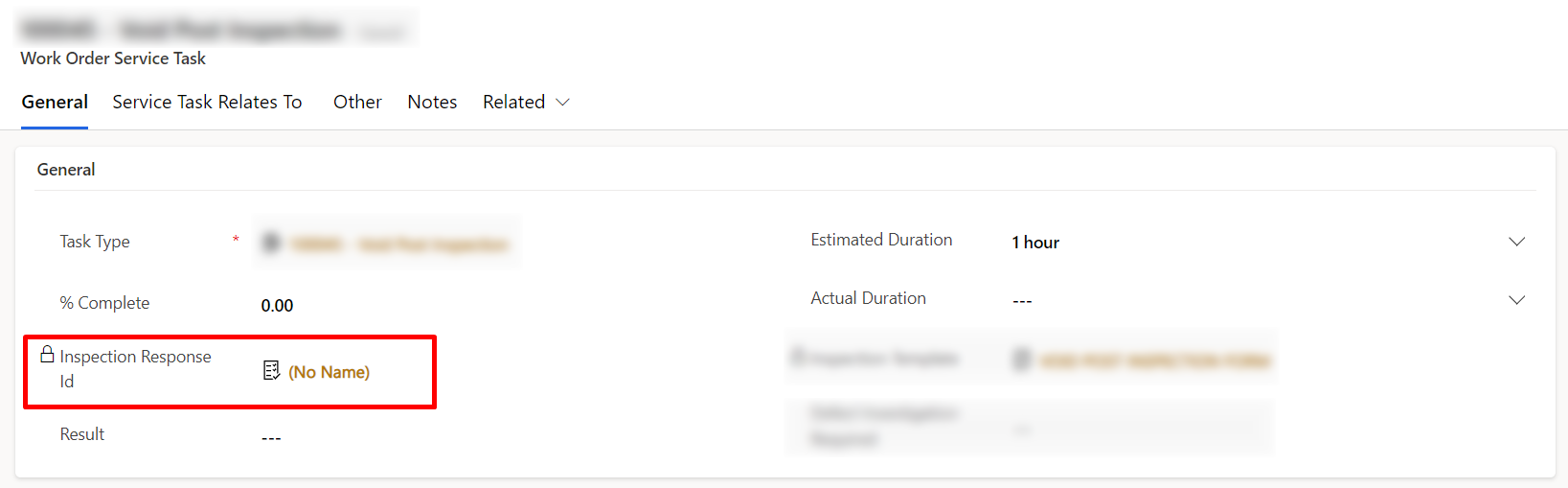
To enable prepopulating questions on an inspection, you need to add the form library CRM.WorkOrderServiceTask.Onload as a trigger. This function is triggered when the form is loaded and waits for the Inspection Response Id to be populated. Once the Id is populated, the function CRM.WorkOrderServiceTask.GetInspectionResponseId is executed to fetch the Inspection Response record.
A while loop is used because it is uncertain when Microsoft will populate the Inspection Response Id field. This method ensures that the Inspection Response Id is always captured.
After acquiring the Inspection Response Id, the CRM.WorkOrderServiceTask.UpdateInspectionResponse function is used to populate the Inspection questions. In the sample code provided below, three questions are populated using JSON. Although the answers are hardcoded in this example, typically, you would need to retrieve your own answers by constructing fetch requests and passing dynamic values into the questions. The JSON data is then passed to the previously fetched Inspection Response.
In addition to prepopulating questions, the code also includes features to improve the user experience, such as a loading screen while the prepopulating code is executing and closing and reopening the form to ensure it works on both desktop and mobile platforms.
Full code below:
if (typeof CRM === 'undefined') CRM = {};
if (typeof CRM.WorkOrderServiceTask === "undefined") CRM.WorkOrderServiceTask = {};
CRM.WorkOrderServiceTask.Onload = async function (executionContext) {
var formContext = executionContext.getFormContext();
var recordId = formContext.data.entity.getId();
if (formContext.getAttribute("msdyn_inspectionresponseid"))
{
if (formContext.getAttribute("msdyn_inspectionresponseid").getValue() === null)
{
var inspectionResponseId = await CRM.WorkOrderServiceTask.GetInspectionResponseId(recordId);
while (inspectionResponseId === null || typeof inspectionResponseId === 'undefined') {
Xrm.Utility.showProgressIndicator("Generating Inspection...");
inspectionResponseId = await CRM.WorkOrderServiceTask.GetInspectionResponseId(recordId);
}
if (inspectionResponseId !== null || typeof inspectionResponseId !== 'undefined') {
CRM.WorkOrderServiceTask.UpdateInspectionResponse(formContext, inspectionResponseId, recordId);
}
}
}
};
CRM.WorkOrderServiceTask.UpdateInspectionResponse = function (formContext, inspectionResponseId, recordId) {
var updatedJson = {
Question1: "Yes",
Question2: "Keys needed",
Question3: "2023-02-08T00:00:00.000Z",
};
var base64EncodedUpdatedJson = btoa(JSON.stringify(updatedJson));
var record = {};
record.msdyn_responsejsoncontent = base64EncodedUpdatedJson;
Xrm.WebApi.updateRecord("msdyn_inspectionresponse", inspectionResponseId, record).then(
function success(result) {
formContext.ui.close();
Xrm.Utility.openEntityForm("msdyn_workorderservicetask", recordId);
Xrm.Utility.closeProgressIndicator();
},
function error(error) {
}
);
};
CRM.WorkOrderServiceTask.GetInspectionResponseId = function (recordId) {
return new Promise(function (resolve, reject) {
Xrm.WebApi.retrieveRecord("msdyn_workorderservicetask", recordId, "?$select=_msdyn_inspectionresponseid_value").then(
function success(result) {
var msdyn_inspectionresponseid = result["_msdyn_inspectionresponseid_value"]; // Lookup
resolve(msdyn_inspectionresponseid);
},
function(error) {
resolve(error);
}
);
});
};
Hi,
Blog is very helpful. I have requirement in the inspection templates.
I have 2 questions as below:
question1 have 3 option set values as 1,2,3
question2 have 3 option set values as 1,2,3
where I have question3 Calculation of values from question1 and question2
if question1 is 1 and question2 is 2 then question3 should be 3
How can i achive this, please let me know.